Number of running and pending solver sessions
When starting a new solver session it is useful to know whether there are other server sessions started on the same server. This article explains how to find out the number of sessions running at an AIMMS PRO server.
Scope
We will discuss two variations on the number of server sessions:
The number of AIMMS PRO sessions actually running.
The number of AIMMS PRO active sessions, i.e., sessions not yet finished.
Obtain number of sessions running
To obtain the number of solver session actually running,
you can use pro::sessionmanager::ListSessionSinceDate
.
We use the following code,
where p_NoRun
contains the number of server sessions actually running.
1p_ret := pro::Initialize();
2if not (p_ret) then return 0; endif;
3
4if pro::GetPROEndPoint() then
5 sp_SinceDate := fnc_CurrentToStringDelta(-24*60*60); ! Assuming jobs older than a day are no longer interesting.
6
7 p_AllUsers := 1 ;
8 if (p_AllUsers) then
9 sp_SessionModelId := "";
10 sp_SessionModelVersion := "";
11 else
12 sp_SessionModelId := pro::ModelName; ! Retrieve sessions for all versions of the model
13 sp_SessionModelVersion := ""; ! don't restrict to a specific ModelVersion
14 endif;
15
16 ! Load raw session list from PROT
17 p_ret := pro::sessionmanager::ListSessionSinceDate(
18 dateStr : sp_SinceDate,
19 SessionList : S_SessionList,
20 ClientQueue : sp_ClientQueue,
21 WorkerQueue : sp_WorkerQueue,
22 CurrentStatus : p_CurrentStatus,
23 CreateTime : sp_CreateTime,
24 userEnvironment : sp_UserEnv,
25 UserName : sp_UserName,
26 proj : sp_Application,
27 clientRef : sp_OriginalCasePath,
28 descr : sp_RequestDescription,
29 proc : sp_RequestProcedure,
30 timeOut : p_RunTimeOut,
31 inputDataVersion : sp_VersionID,
32 outputDataVersion : sp_ResponseVersionID,
33 logFileVersion : sp_MessageLogVersionID,
34 ErrorMessage : sp_ErrorMessage,
35 modelStatus : p_ActiveStatus,
36 ErrorCode : p_ErrorCode,
37 AllUsers : p_AllUsers,
38 projectID : sp_SessionModelId,
39 projectVersion : sp_SessionModelVersion);
40 if not (p_ret) then return 0; endif;
41 p_NoCreated := count( i_sess | p_CurrentStatus(i_sess) = pro::PROTS_CREATED );
42 p_NoQueued := count( i_sess | p_CurrentStatus(i_sess) = pro::PROTS_QUEUED );
43 p_NoInitializing := count( i_sess | p_CurrentStatus(i_sess) = pro::PROTS_INITIALIZING );
44 p_NoReadyToRun := count( i_sess | p_CurrentStatus(i_sess) = pro::PROTS_READY );
45 p_NoRun := count( i_sess | p_CurrentStatus(i_sess) = pro::PROTS_RUNNING );
46else
47 p_ret := 0 ;
48endif;
49
50return p_ret ;
Obtaining number of active sessions
Next, to find the active sessions we’ll aggregate number of unfinished sessions.
Based on the above, aggregating the number of unfinished sessions is simple:
1p_DerivedActiveJobs(ep_obs) :=
2 p_ObservedCreatedJobs( ep_obs ) +
3 p_ObservedQueuedJobs( ep_obs ) +
4 p_ObservedInitializingJobs( ep_obs ) +
5 p_ObservedReadyToRunJobs( ep_obs ) +
6 p_ObservedRunningJobs( ep_obs ) ;
Example projects
You can download two example apps to try out the code yourself:
Start several jobs with
FlowShopMultipleSolves
Show active jobs with
CountRunningJobs
To experiment with these apps, you should download and publish them both on AIMMS PRO. Start both apps, and press the start button of both apps.
You’ll then see how CountRunningJobs
monitors multiple jobs:
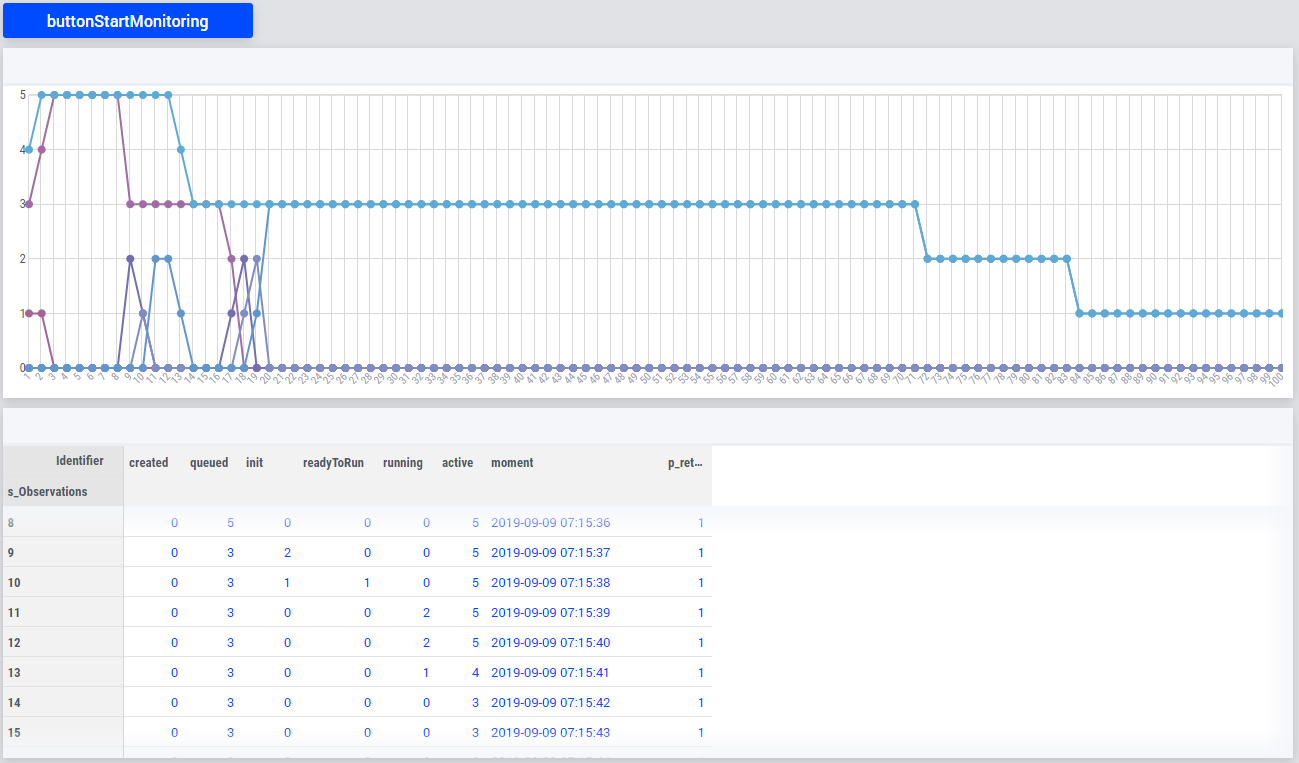
You can copy the procedure pr_CountRunningJobs
(in the app CountRunningJobs
) to
determine the number of running jobs or the number of active jobs in your own application.
Important
It is possible that two users at the same time query the number of running jobs, which return 0, and then submit a job. In this case, there may still be one job waiting for the other.
To detect such cases, the number of active jobs is one more than the number allowed to run in parallel.
To detect whether the waiting job is your job, you can test for pro::session::CurrentSessionStatus
.
You can cancel the waiting job as explained in Interrupt the solver session.