Warning
Please note that the documentation you are currently viewing is for an older version of our technology. While it is still functional, we recommend upgrading to our latest and more efficient option to take advantage of all the improvements we’ve made. See DEX.
Retrieve Geographic Coordinates with Google Maps API
In this article, we will learn how to obtain coordinates using a HTTP request. We’ll use the Geocoding API from Google Maps, which returns coordinates for a given location.
Prerequisites
Before we begin, there are a couple things you’ll need to have in place.
Install the HTTP library, please read Adding the HTTP Client Library.
Get a Google API key. Get one from Google Maps Platform: Get an API Key.
Example Project
You can download the example AIMMS project here:
Informations About the Request
Scrolling the Google Maps API documentation, we can learn precious information about how to formulate our request. The Authentication is made using an API key and the URL should take the following shape:
To provide output in a format compatible with AIMMS, we will specify XML rather than JSON in our request. We can also find the list of required parameters for the request:
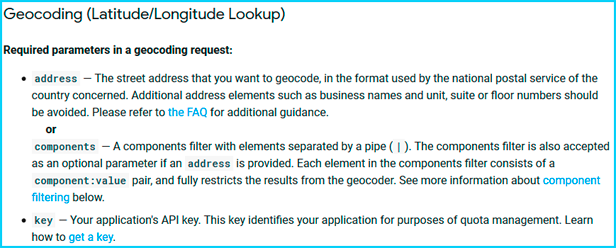
Here, we will use the key and the address parameters. The Google Maps API documentation, lists several optional parameters to further specify your request.
Creating the Request
To create the request, the code is as follows:
1SP_responseFile:="OutputCoordinates.xml";
2SP_key:="YOUR_API_KEY";
3SP_location:="AIMMS Haarlem";
4SP_requestparameters:= DATA{key,address};
5SP_requestparameters['key']:=SP_key;
6SP_requestparameters['address']:=SP_location;
7web::query_format(SP_requestparameters,SP_formattedparameters);
8
9SP_URL:="https://maps.googleapis.com/maps/api/geocode/xml?"+SP_formattedparameters;
10web::request_create(SP_requestId);
11web::request_setMethod(SP_requestId,"GET");
12web::request_setURL(SP_requestId,SP_URL);
13web::request_setResponseBody(SP_requestId,'File',SP_responseFile);
14web::request_invoke(SP_requestId,P_responsecode);
You’ll also need the following AIMMS identifiers:
Parameter P_Lat;
Parameter P_Long;
StringParameter SP_responseFile;
Set S_reqpar {
Index: rp;
InitialData: DATA{key,address};0001
}
StringParameter SP_requestparameters {
IndexDomain: rp;
}
StringParameter SP_URL;
StringParameter SP_requestId;
Parameter P_responsecode;
StringParameter SP_key;
StringParameter SP_location;
StringParameter SP_formattedparameters;
Note
Learn more about the basics of HTTP request in AIMMS in Extract XML File from a Server with the HTTP Library.
Learn more about HTTP requests for Google APIs in Retrieve Geographic Data with Google Maps API.
Retrieving and Mapping XML Data
We want to know the location of the AIMMS office in Haarlem. After executing the request procedure, we get in the SP_responseFile
direction the XML answer from the API.
Using the XSD generator from Freeformatter.com, we can get the XSD file required to use the AIMMS XML schema Mapping tool. Let’s put this XSD file in the root of our project, with the name “googlecoord.xsd
”.
The XML Schema mapping tool generates an AXM file from an XSD file to map the data into AIMMS identifiers.
For a tutorial about XML mapping, read Extract Data from an XML File.
We want to do the following mapping:
Geometry/location/lat
maps-toP_lat
Geometry/location/lng
maps-toP_long
put every read-filter attribute to 0 except for the objects used
(lat,lng,geometry,location,result)
Then, by using the READXML
method, we can extract the coordinates we want into AIMMS.
READXML(SP_responseFile,"googlecoord.axm");
Complete the XSD
After completing these steps, you may expect that modifying the SP_location
gives us access to the coordinates of any location we want.
But, if you change the value of SP_location
to Amsterdam, you’ll have the following error message after executing the complete procedure:

The reason is that the Google API sends back an XML file that has a slightly different shape depending on the location you’re asking for; some elements are added or deleted. This means that some elements of the XML file from the request are not in the XSD file, which causes an error.
To solve this problem, we would need need to know all possible XML answer formats from this API, which Google doesn’t provide. Therefore we can only expand our current XSD file with the missing information after each error encountered.
The error refers to a ‘bound’ element missing from our current XSD file. Let’s check where it is.
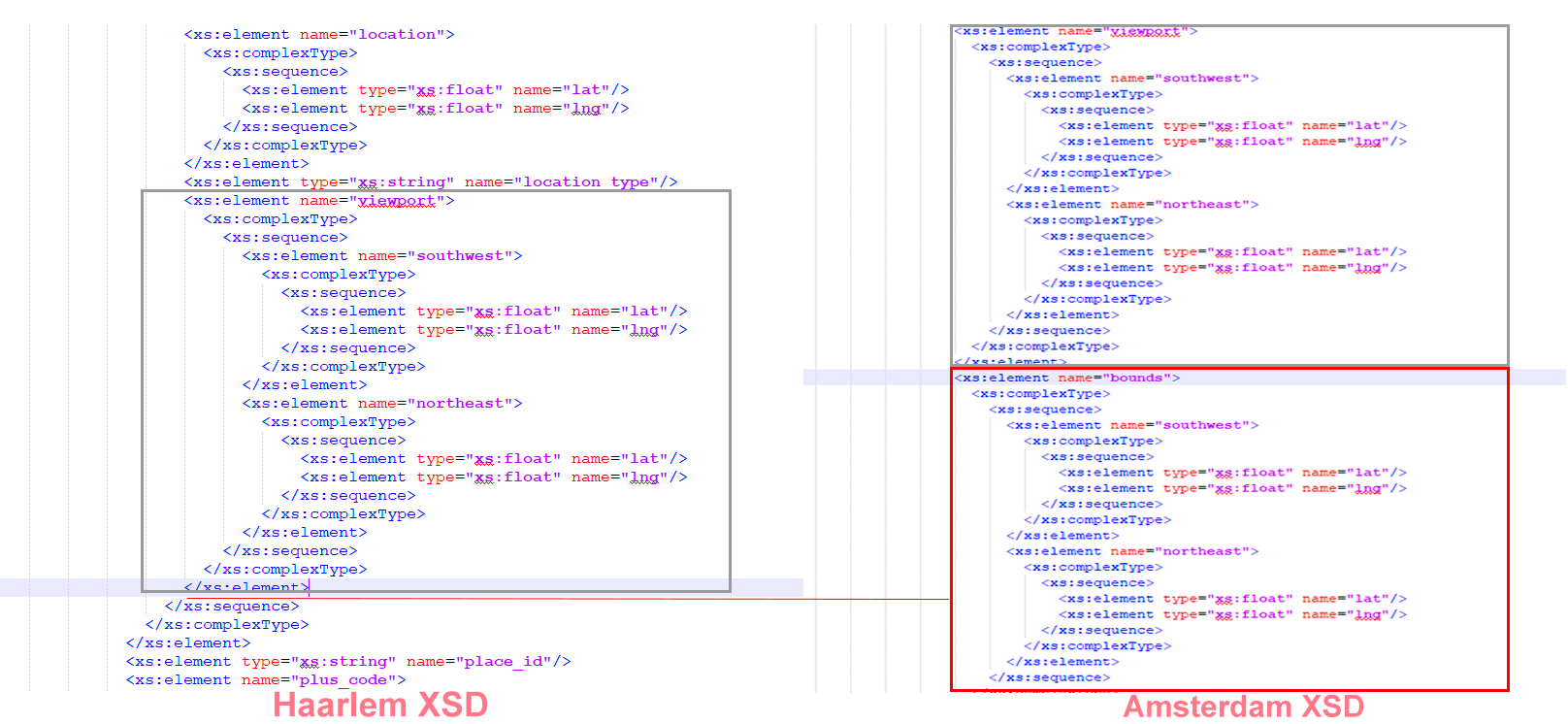
The bound
is set after the viewport
node in the Amsterdam XSD file, so let’s copy/paste the block in the original XSD file.
Then, again using the XML schema mapping tool to set the bound
read-filter attribute to 0
and executing the procedure, we can get the proper coordinates.
We’re providing an XSD file to get you started: googlecoord.zip
However, this file may be incomplete, so be prepared to make your own additions to the XSD file while using this API.