Warning
Please note that the documentation you are currently viewing is for an older version of our technology. While it is still functional, we recommend upgrading to our latest and more efficient option to take advantage of all the improvements we’ve made.
Extract Photos Using the Flickr API
In this article, we’ll learn how to use the Flickr REST API.
The process to deal with a REST API in AIMMS is very similar, however you can find details of the differences in AIMMS Documentation: HTTP Client Library. Here, we use a concrete example of this process to supplement the documentation.
Use Case
This use case is inspired by an idratherbewriting tutorial.
Flickr is an image and video hosting social network with a database of millions of photos. Our mission will be to extract the photos from a Flickr gallery.
Prerequisites
Before you begin, make sure you have done the following:
Install the AIMMS HTTP Client Library
Obtain an API Key
Example Project
You can download the example project for this tutorial from the link below:
Required Information
Information about the URL format where we can address our request and the authentication system to use can be found in Flickr API documentation, specifically the sections labeled URLs, Overview and User Authentication.
Note
According to Flickr’s documentation, the main authentication system used for this API is a complex OAuth protocol, but some methods can still be used with a simple API key. We’ll use the API key method here.
The request format is specified as a URL endpoint followed by these parameters:
method
(REQUIRED) - specify the calling methodapi_key
(REQUIRED) - specify your API Keyformat
(optional) - specify a response format
The arguments, responses, and error codes for each method are listed on the method’s spec page, found on the Flickr API index page.
To download an image using a GET
request, we need a photo URL.
To access a photo, we need the following:
the farm_id
(optional - the server will provide it if you don’t)the server_id
id
secret
The URL format is specified in the Flickr documentation:
https://farm{farm-id}.staticflickr.com/{server_id}/{id}_{secret}.jpgTo get this information about particular photos, we can use the method flickr.galleries.getPhotos to return the information required for creating the individual photo URLs.
First, we need the id
of the gallery. We’ll use the flickr.urls.lookupGallery to get that.
Gathering Required Information
The process to gather the required information can be summarized as follows:
Create a request to obtain the gallery
id
using flickr.urls.lookupGalleryCreate a request to obtain gallery photos using flickr.galleries.getPhotos
Construct the photos URLs and do a GET request to obtain the files.
Getting the Gallery id
The code for this request is the following one.
1SP_responseFile:="Output.xml";
2 SP_APIkey:="Your_api_key";
3SP_requestparameters:={
4 'method': "flickr.urls.lookupGallery",
5 'api_key': SP_APIkey,
6 'url': "https://www.flickr.com/photos/flickr/galleries/72157647277042064/"
7};
8web::query_format(SP_requestparameters,SP_formattedparameters);
9SP_URL:="https://www.flickr.com/services/rest/?"+SP_formattedparameters;
10
11!set the request
12web::request_create(SP_requestId);
13web::request_setMethod(SP_requestId,"GET");
14web::request_setURL(SP_requestId,SP_URL);
15web::request_setResponseBody(SP_requestId,'File',SP_responseFile);
16web::request_invoke(SP_requestId,P_responsecode);
17
18!extract data from the XML.
19READXML("Output.xml","GetGallery.axm");
The HTTP Request
For details about how to formulate an HTTP request, please follow the article Extract XML File from a Server with the HTTP Library.
Our goal is to use the flickr.urls.lookupGallery
method from the API using a GET request and to extract the gallery id
from the answer file.
Let’s check what the Flickr documentation has to tell us about this method.
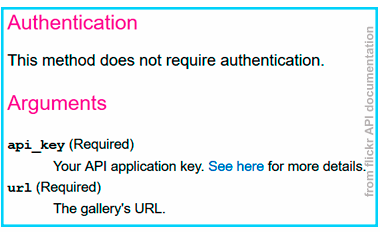
The request requires two arguments, API key URL. The URL of the gallery is as follows: https://www.flickr.com/photos/flickr/galleries/72157647277042064/
Note
Using the example project, you can extract photos from any gallery by setting the SP_url
to the URL of the wanted gallery.
For this request, you’ll need several objects:
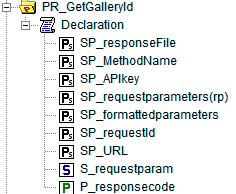
1Set S_requestparam {
2 Index: I_rp;
3 InitialData: {
4 DATA{api_key,method,url};
5 }
6}
7StringParameter SP_requestId {
8
9}
10StringParameter SP_requestparameters {
11 IndexDomain: I_rp;
12}
13Parameter P_responsecode {
14
15}
16StringParameter SP_APIkey {
17
18}
19StringParameter SP_MethodName{
20
21}
22StringParameter SP_URL {
23
24}
25StringParameter SP_responsefile{
26
27}
28StringParameter SP_formattedparameters {
29
30}
We can specify the direction for the output file using the SP_OutputFile
string parameter.
SP_responseFile:="Output.xml";
The parameters of the request must be put at the end of the endpoint URL https://www.flickr.com/services/rest/?
after being formatted by the web::query_format
method.
1SP_requestparameters:={
2 'method': "flickr.urls.lookupGallery",
3 'api_key': SP_APIkey,
4 'url': "https://www.flickr.com/photos/flickr/galleries/72157647277042064/"
5};
6
7web::query_format(SP_requestparameters,SP_formattedparameters);
8SP_URL:="https://www.flickr.com/services/rest/?"+SP_formattedparameters;
We can then set our request as usual.
1web::request_create(SP_requestId);
2web::request_setMethod(SP_requestId,"GET");
3web::request_setURL(SP_requestId,SP_URL);
4web::request_setResponseBody(SP_requestId,'File',SP_responseFile);
5web::request_invoke(SP_requestId,P_responsecode);
We should now have our XML file accessible at the root of the project, or wherever it is specified to go.
Extracting Data
For details of how to extract data from an XML file in AIMMS, check this step-by-step article: Extract Data from an XML File.
With this new XML file, we can now create an XSD file in order to use the XML schema mapping tool from AIMMS.
To generate this XSD file, you can use an online generator such as the one provided by FreeFormatter. Once it’s obtained, just put the XSD file at the root of your project.
Now create a string parameter SP_GalleryID
made for containing the gallery ID information and using the XML schema mapping tool, map it to the rsp/gallery/id
element of the XML file (not the rsp/gallery/Gallery_id
element).
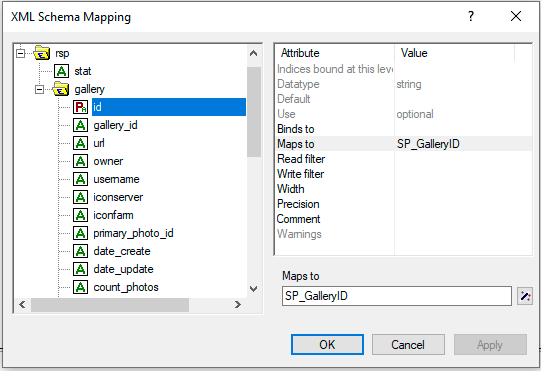
Warning
Be sure to set every read-filter
attribute of unused elements from the XML schema mapping tools to “0”.
Finally, by using the READXML
method, we can get the gallery ID we’re searching for.
READXML("Output.xml","NAME_OF_YOUR_XSD.axm");
Getting Photo Information
We now want to create another procedure to get all the id
information we need about the gallery photos.
For that, we’ll use the flickr.galleries.getPhotos
method from the Flickr API. The code for this procedure is as follows:
1SP_responseFile:="Output2.xml";
2SP_APIkey:= "Your_api_key";
3SP_MethodName:="flickr.galleries.getPhotos";
4SP_requestparameters:= {
5 'method' : SP_MethodName,
6 'api_key' : SP_APIkey,
7 'gallery_id' : SP_GalleryID
8};
9
10web::query_format(SP_requestparameters,SP_formattedparameters);
11SP_URL:="https://www.flickr.com/services/rest/?"+SP_formattedparameters;
12web::request_create(SP_requestId);
13web::request_setMethod(SP_requestId,"GET");
14web::request_setURL(SP_requestId,SP_URL);
15web::request_setResponseBody(SP_requestId,'File',SP_responseFile);
16web::request_invoke(SP_requestId,P_responsecode);
17READXML("Output2.xml","NAME_OF_YOUR_XSD.axm");
Set the HTTP Request
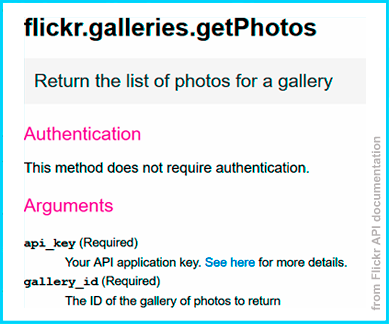
This request takes the parameters api_key
and gallery_id
, and we want from the answer the farm
ID, the server ID
, the ID
and the secret
for each photo in the gallery.
But before extracting these, we need to get the XML file containing this information from an HTTP request.
The process is almost the same as in the last request, the only thing changing here is the DATA of the set S_requestparam
and the method used:
You need to create these objects:
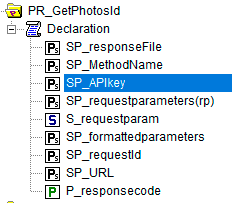
1Set S_requestparam {
2 Index: I_rp;
3 Definition: {
4 DATA{api_key,method,gallery_id};
5 }
6 }
7StringParameter SP_requestId {
8
9}
10StringParameter SP_requestparameters {
11 IndexDomain: I_rp;
12}
13Parameter P_responsecode {
14
15}
16StringParameter SP_APIkey {
17}
18StringParameter SP_MethodName{
19
20}
21StringParameter SP_URL {
22
23}
24StringParameter SP_responsefile{
25
26}
27StringParameter SP_formattedparameters {
28
29}
Then execute this code:
1SP_responseFile:="Output2.xml"; 2SP_APIkey:= "Your_api_key"; 3SP_MethodName:="flickr.galleries.getPhotos"; 4SP_requestparameters:= { 5 'method' : SP_MethodName, 6 'api_key' : SP_APIkey, 7 'gallery_id' : SP_GalleryID 8}; 9 10web::query_format(SP_requestparameters,SP_formattedparameters); 11SP_URL:="https://www.flickr.com/services/rest/?"+SP_formattedparameters; 12web::request_create(SP_requestId); 13web::request_setMethod(SP_requestId,"GET"); 14web::request_setURL(SP_requestId,SP_URL); 15web::request_setResponseBody(SP_requestId,'File',SP_responseFile); 16web::request_invoke(SP_requestId,P_responsecode);
You should now have access to the XML answer file in the direction SP_responseFile
, and you can generate your second XSD file or download it
.
Extracting Data
Before extracting the data from the XML file using the AIMMS XML schema mapping tool, you need to create objects to contain this information:
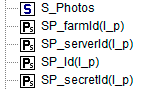
Set S_Photos {
Index: I_p;
}
StringParameter SP_farm(I_p) {
IndexDomain: I_p;
}
StringParameter SP_server(I_p) {
IndexDomain: I_p;
}
StringParameter SP_id(I_p) {
IndexDomain: I_p;
}
StringParameter SP_secret(I_p) {
IndexDomain: I_p;
}
Using the XML mapping tool, you then need to make the following mapping:
rsp/photos/photo/title
binds-toS_Photos
.rsp/photos/photo/id
maps-toSP_Id
.rsp/photos/photo/farm
maps-toSP_farm
.rsp/photos/photo/server
maps-toSP_server
.rsp/photos/photo/secret
maps-toSP_secret
.
Then execute:
READXML("Output2.xml","NAME_OF_YOUR_XSD.axm");
You should now have a set S_Photos
containing photo titles of the gallery and having for parameters the id
, farm
id, server
id and secret
of a photo.
Getting the Photos
We know from the Flickr API Documentation: URLs the format a photo URL must have. This URL is different from the one displayed on your browser when you select the photo. This isn’t the URL of the page where we can find the photo but the URL of the photo itself.
https://farm{farm-id}.staticflickr.com/{server_id}/{id}_{secret}.jpgNow we’ll set a GET request to the URL corresponding to each photo contained in the gallery, to obtain the photos. For that, we need some new objects:
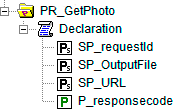
StringParameter SP_requestId {
}
Parameter P_responsecode {
}
StringParameter SP_URL {
}
StringParameter SP_responsefile{
}
The code of this procedure is as follows:
1for p in S_Photos Do
2 !set direction for the photos
3 SP_OutputFile:="MainProject/WebUI/resources//images/"+SP_id(I_p)+".jpg";
4 !create URLs
5 SP_URL:="https://farm"+SP_farmId(p)+".staticflickr.com/"+SP_serverId(p)+"/"+SP_Id(p)+"_"+SP_secretId(p)+".jpg";
6 !send request
7 web::request_create(SP_requestId);
8 web::request_setMethod(SP_requestId,"GET");
9 web::request_setURL(SP_requestId,SP_URL);
10 web::request_setResponseBody(SP_requestId,'File',SP_OutputFile);
11 web::request_invoke(SP_requestId,P_responsecode);
12endfor;
The choice to set the names of photo files using the SP_id(I_p)
parameter is arbitrary. The result is that every file name is the ID of the concerned photo in Flickr. (If you chose to use title of photos, for example, unsupported special characters may be included.)
The choice of the destination MainProject/WebUI/resources/images/
refers to the use of WebUI Image Widget.
Congratulations, we finally reached our goal!
Now we can use the photos in AIMMS:
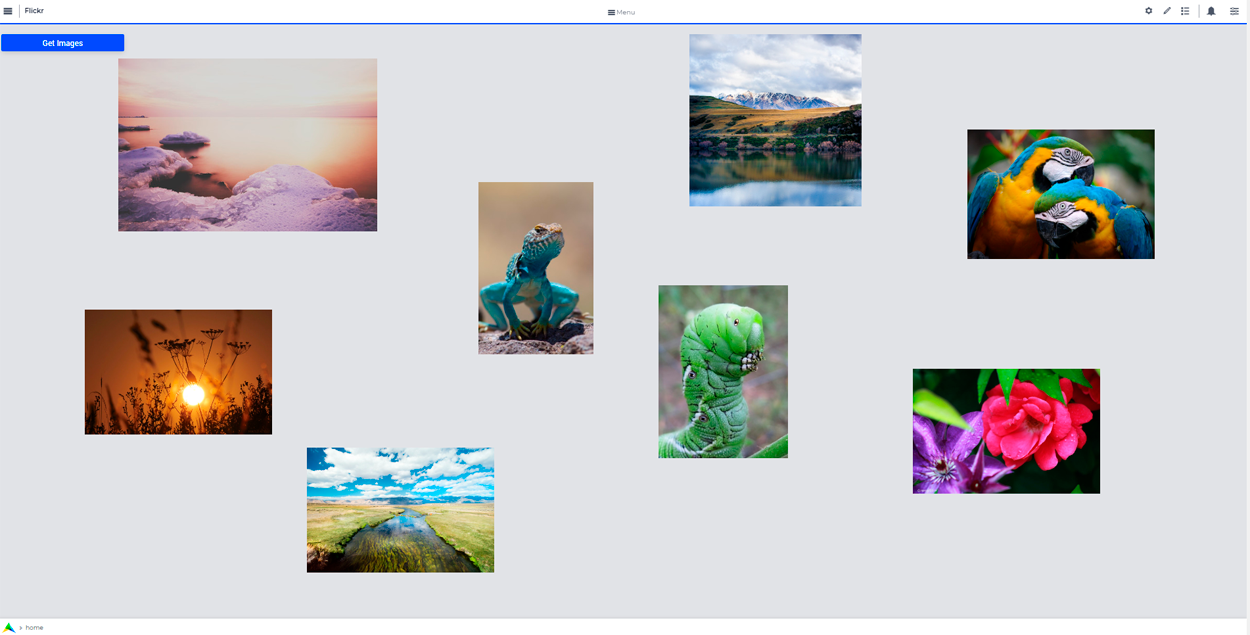
Further Information
The Flickr API also allows you to search for photos using tags with the flickr.photos.search method.
It will then send you back a list of photos identified by those tags with all the IDs you need to recreate their URL. And by mapping the data into AIMMS and making a GET request to the newly created URLs, you can get the photos. You will find the related code in the example project.
Note
Please note that you can only specify 20 tags at the same time, and the answer will contain only one page of results (max 500 photos).