Zoom and Scroll in a Gantt Chart
This article presents an elegant trick to use one Gantt Chart to control the scrolling and zooming of another Gantt Chart. The result is shown in the image below.
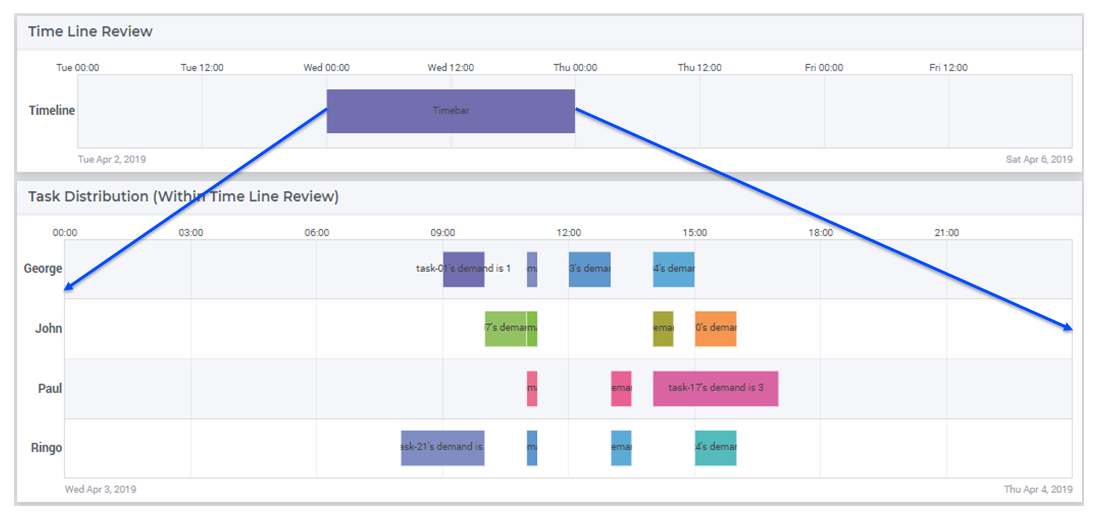
Creating a single bar
The Gantt chart used to create the scrolling and zooming functionality is only one bar, but we still have sets to cover the resources (one) and jobs (one), parameters for start and duration, element parameters to denote this one bar, and two string parameters to specify the viewport.
In our example this is achieved with the code below:
1Set s_TimebarResourceSet {
2 Index: i_tb_res;
3 Parameter: ep_tb_selectedResource;
4 Definition: data {Timeline};
5}
6Set s_TimebarJobSet {
7 Index: i_tb_job;
8 Parameter: ep_tb_selectedJob;
9 Definition: data {Timebar};
10}
11StringParameter sp_TimebarDuration_text {
12 IndexDomain: (i_tb_res,i_tb_job);
13}
14Parameter p_TimebarTimeStart {
15 IndexDomain: (i_tb_res,i_tb_job);
16 Unit: hour;
17}
18Parameter p_TimebarDuration {
19 IndexDomain: (i_tb_res,i_tb_job);
20 Unit: hour;
21 webui::ItemTextIdentifier: sp_TimebarDuration_text;
22}
23StringParameter sp_TimebarViewportStart;
24StringParameter sp_TimebarViewportEnd;
These identifiers are initialized in the following procedure:
1Procedure pr_InitializeTimebarGanttChart {
2 Body: {
3 ! The view port of the Timebar Gantt Chart should allow for the maximum
4 ! view port of the actual Gantt Chart.
5 sp_today := CurrentToString("%c%y-%m-%d");
6 sp_yesterday := MomentToString(
7 Format : "%c%y-%m-%d",
8 unit : [day],
9 ReferenceDate : sp_today,
10 Elapsed : -1[day]);
11 sp_twoDaysAfterTomorrow := MomentToString(
12 Format : "%c%y-%m-%d",
13 unit : [day],
14 ReferenceDate : sp_today,
15 Elapsed : 3[day]);
16
17 sp_TimebarViewportStart := sp_yesterday + " 00:00";
18 sp_TimebarViewportEnd := sp_twoDaysAfterTomorrow + " 00:00";
19
20 ! There is only one resource/job so the element parameters are always set to this one.
21 ep_tb_selectedResource := first( s_TimebarResourceSet );
22 ep_tb_selectedJob := first( s_TimebarJobSet );
23
24 ! The start/duration of the only job in the timebar Gantt Chart
25 ! should be initialized the same as the controlled Gantt Chart Viewport was initialized.
26 ! In this example we assume for the sake of simplicity that the second day is ok.
27 p_TimebarTimeStart( ep_tb_selectedResource, ep_tb_selectedJob) := 1[day];
28 p_TimebarDuration( ep_tb_selectedResource, ep_tb_selectedJob) := 1[day];
29 sp_TimebarDuration_text( ep_tb_selectedResource, ep_tb_selectedJob) := "Timebar";
30 }
31 StringParameter sp_today;
32 StringParameter sp_yesterday;
33 StringParameter sp_twoDaysAfterTomorrow;
34}
Defining the viewport
The beginning of the timebar defines the beginning of viewport of the lower Gantt Chart (sp_SelectedViewPortStart
),
and similarly, the end the timebar defines the end of the viewport of the lower Gantt Chart (sp_SelectedViewPortEnd
).
These two string parameters are defined as follows:
1Parameter bp_useUTCforSelectedViewport {
2 Range: binary;
3}
4ElementParameter ep_timezoneForSelectedViewport {
5 Range: AllTimeZones;
6 Definition: {
7 if bp_useUTCforSelectedViewport then
8 'UTC'
9 else
10 webui::WebApplicationTimeZone
11 endif
12 }
13}
14StringParameter sp_SelectedViewPortStart {
15 Definition: {
16 MomentTostring("%c%y-%m-%d %H:%M%TZ(ep_timezoneForSelectedViewport)",
17 [hour], sp_TimebarViewportStart,
18 p_TimebarTimeStart(ep_tb_selectedResource,ep_tb_selectedJob));
19 }
20}
21StringParameter sp_SelectedViewPortEnd {
22 Definition: {
23 MomentTostring("%c%y-%m-%d %H:%M%TZ(ep_timezoneForSelectedViewport)",
24 [hour], sp_TimebarViewportStart,
25 p_TimebarTimeStart(ep_tb_selectedResource,ep_tb_selectedJob)+
26 p_TimebarDuration(ep_tb_selectedResource,ep_tb_selectedJob));
27 }
28}
Here bp_useUTCforSelectedViewport
is initialized using the following code:
1block
2 bp_ogv := OptionGetValue("Use UTC forcaseandstartenddate",
3 p_optLow, p_optCur, p_optDef, p_optUpp );
4 if p_optCur then
5 bp_useUTCforSelectedViewport := 1 ;
6 else
7 bp_useUTCforSelectedViewport := 0 ;
8 endif ;
9endblock ;
Example project
The example can be downloaded below.