Using AIMMS Services with a VBA Client
AIMMS is an elaborate tool for modeling optimization problems. Excel is a popular tool to model business problems. This article is about leveraging the power of AIMMS in EXCEL via HTTP requests.
Preparation
Excel comes with Visual Basic for Applications (VBA) permitting via the
WinHttp.WinHttpRequest.5.1
library to leverage REST API services.
Preparing to create a VBA Client of your own using AIMMS Rest API Tasks, you will have to:
Enable some libraries, via the Visual Basic Editor (
Alt + F11
), , most importantly:Microsoft WinHTTP Services, version 5.1
This library facilitates HTTP requests.
-
Amongst others this library facilitates dictionaries.
Download and install The VBA JSON library.
Workbook
In this section, a simple example of an end user interface of a VBA app that deploys the CountTheStars interface as implemented by an AIMMS app.
FrontEnd
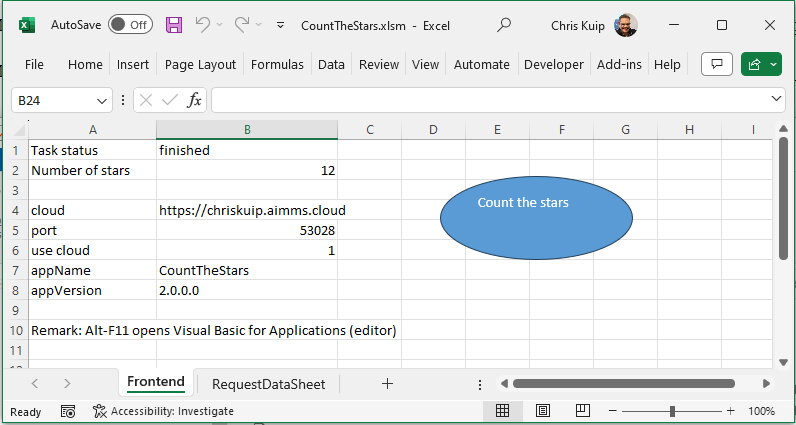
Cell
B1
will contain the state of the request as it executes.Cell
B2
will contain the number of stars upon finishing the request.Cells
B4-B8
contain some additional info.The button labeled “Count the stars” will execute the
CountTheSTars
service in the AIMMS app.
RequestDataSheet
This sheet contains sample input data.
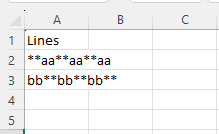
VBA
There are four steps, each detailed in separate subsection
Preparation
Via the subroutine getConfig
the configuration to be tested is read in following
the conventions set out in Conventions Used in the Examples Provided
Initiate
In this sub section, we’ll handle submitting a request for executing a task using VBA.
The code to initiate an AIMMS task is:
1Sub RequestCountTheStars_usingWinHTTP51(apiURL As String, apiKey As String, useCloud As Integer, JsonString As String, ByRef taskId As String)
2'
3' Use the WinHTTP51 library to actually do a POST on the apiURL for running a task.
4'
5
6 ' Open the connection and set the method to POST
7 Http.Open "POST", apiURL, False
8
9 ' Set the request headers
10 If useCloud Then
11 Http.SetRequestHeader "apikey", apiKey
12 End If
13 Http.SetRequestHeader "Content-Type", "application/json"
14
15 ' Send the request
16 Http.Send JsonString
17
18 If Http.Status = 200 Then
19 ' Request successful
20 Dim JsonRT As String
21 JsonRT = Http.responseText
22 Dim Parsed As Object
23 Set Parsed = JsonConverter.ParseJson(JsonRT)
24 taskId = Parsed("id")
25 Else
26 Debug.Print "RequestCountTheStars(): Failure: " & Http.Status & " " & Http.StatusText
27 End If
28
29End Sub
Here the apiURL
is obtained via the subroutine DetURLTaskPostRequest
,
following Development Architecture of an AIMMS Service for constructing url_submit
.
Monitor
The code to monitor the task is:
1Sub PollOnce_usingWinHTTP51(apiURL As String, apiKey As String, useCloud As Integer, ByRef taskState As String)
2'
3' Use the WinHTTP51 library to do a get on the running task, to obtain a task status.
4'
5
6 ' Open the connection and set the method to POST
7 Http.Open "GET", apiURL, False
8
9 ' Set the request headers
10 If useCloud Then
11 Http.SetRequestHeader "apikey", apiKey
12 End If
13
14 ' Send the request
15 Http.Send
16
17 If Http.Status = 200 Then
18 ' Request successful
19 Debug.Print Http.responseText
20 Dim JsonRT As String
21 JsonRT = Http.responseText
22 Debug.Print "PollOnce_usingWinHTTP51, response is: ", JsonRT
23 Dim Parsed As Object
24 Set Parsed = JsonConverter.ParseJson(JsonRT)
25 taskState = Parsed("state") ' Set output argument this procedure
26 Else
27 Debug.Print "PollOnce_usingWinHTTP51, Failure: " & Http.Status & " " & Http.StatusText
28 End If
29
30End Sub
Here the apiURL
is obtained via the subroutine PollOnceDetermineURL
,
following Development Architecture of an AIMMS Service for constructing url_poll
.
Receive Result
The code to receive the result is:
1Sub ReceiveResponse_usingWinHTTP51(apiURL As String, apiKey As String, useCloud As Integer, ByRef noStars As Integer)
2'
3' Do a GET on on the URL for receiveing the response of a task, thus finally getting the results of the task.
4'
5 ' Open the connection and set the method to POST
6 Http.Open "GET", apiURL, False
7
8 ' Set the request headers
9 If useCloud Then
10 Http.SetRequestHeader "apikey", apiKey
11 End If
12
13 ' Send the request
14 Http.Send
15
16 If Http.Status = 200 Then
17 ' Request successful
18 Dim JsonRT As String
19 JsonRT = Http.responseText
20 Dim Parsed As Object
21 Set Parsed = JsonConverter.ParseJson(JsonRT)
22 noStars = Parsed("count")
23 Debug.Print "ReceiveResponse_usingWinHTTP51(): Received response, no stars = ", noStars
24 Else
25 Debug.Print "ReceiveResponse_usingWinHTTP51(): Failure: " & Http.Status & " " & Http.StatusText
26 End If
27
28End Sub
Here the apiURL
is obtained via the subroutine ReceiveResponseDetermineURL
,
following Development Architecture of an AIMMS Service for constructing url_response
.
References
The VBA JSON library
Note
This article also provides an alternative for the deprecation of aimmscom
.
Using AIMMS as an optimization tool inside an Excel workbook used to be facilitated via the Microsoft COM interface, but is now deprecated, see also Deprecation Table .